JavaScript vs. TypeScript: The Future of Typed JavaScript
Shubham Prakash
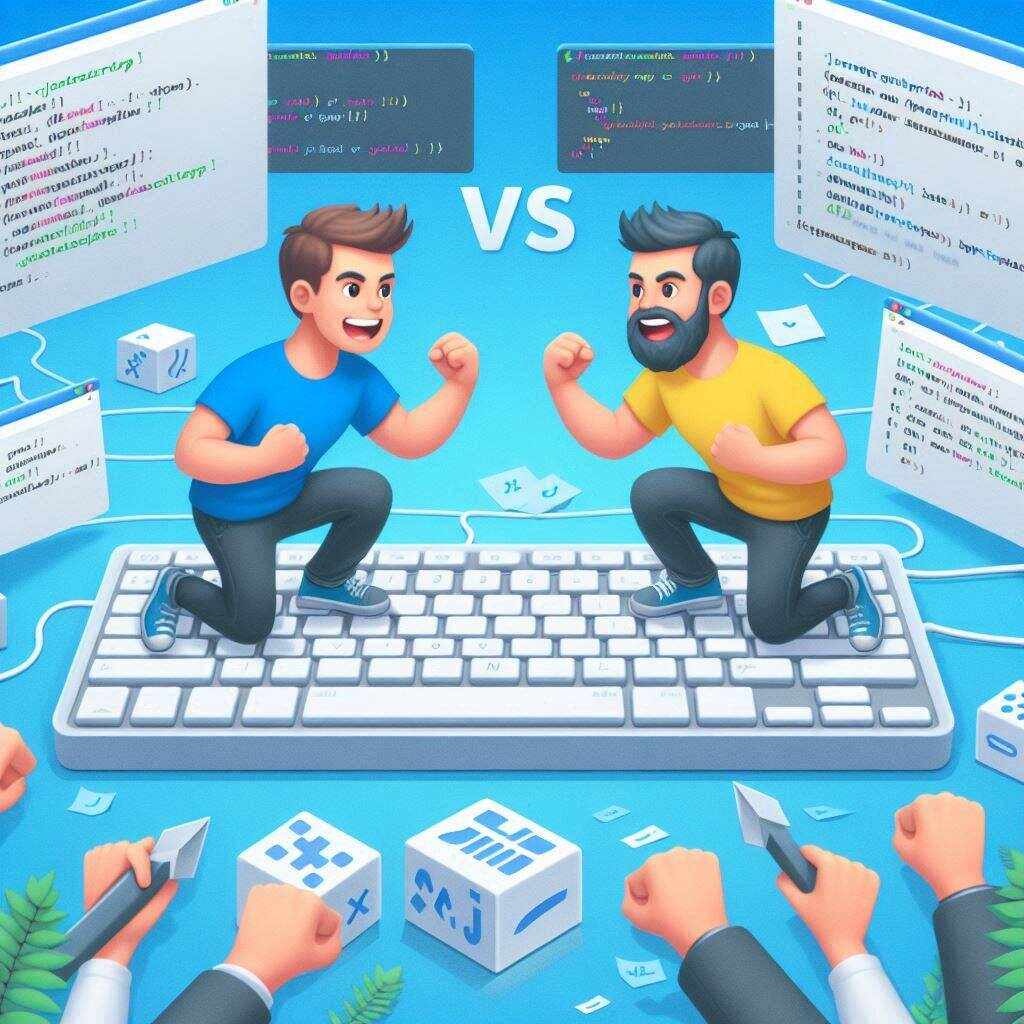
Introduction
JavaScript has been the backbone of web development for decades, enabling developers to create dynamic and interactive websites. However, as web applications become increasingly complex, JavaScript’s loose typing can sometimes lead to runtime errors and difficult-to-maintain codebases. This is where TypeScript, a typed superset of JavaScript, comes into play.
TypeScript has quickly become the preferred choice for many developers and companies in 2024 due to its static typing, enhanced tooling support, and growing ecosystem. In this blog, we'll explore why TypeScript is gaining traction, how it enhances JavaScript development, and how you can transition your existing JavaScript codebase to TypeScript.
1. What is TypeScript?
TypeScript is a superset of JavaScript developed by Microsoft that adds optional static typing to the language. This means that TypeScript builds on JavaScript by adding new features, such as type annotations, interfaces, and compile-time type checking, while retaining full compatibility with JavaScript code.
Key Features of TypeScript:
- Static Typing: TypeScript uses type annotations to define the expected type of variables, functions, and objects, which helps catch errors during development rather than at runtime.
- Type Inference: Even without explicit type annotations, TypeScript can often infer the type based on the assigned value.
- Advanced Tooling and IDE Support: TypeScript offers better autocompletion, navigation, and refactoring tools in IDEs like VS Code, which significantly boosts developer productivity.
- ESNext Support: TypeScript compiles down to plain JavaScript, meaning it can target different JavaScript versions (ES5, ES6, ESNext) and incorporate future ECMAScript features before they are natively supported by browsers.
2. Why Choose TypeScript over JavaScript?
a) Enhanced Code Quality and Maintainability
One of the main advantages of TypeScript is its ability to catch errors at compile time. By enforcing types, TypeScript helps developers identify bugs before they reach production. This is particularly useful in large codebases where changes in one part of the application can have unforeseen consequences elsewhere.
b) Improved Developer Experience
TypeScript’s strong typing system provides better autocompletion and code navigation in modern IDEs. It helps developers quickly understand the codebase, reducing cognitive load and making collaboration easier. Tools like IntelliSense in Visual Studio Code offer smart suggestions, quick fixes, and inline type information, greatly enhancing the developer experience.
c) Scalability for Large Projects
TypeScript’s type system and modular architecture make it easier to scale applications. In large teams, where multiple developers contribute to the same codebase, TypeScript helps enforce consistency and reduces the risk of errors. The explicit typing system ensures that developers are aware of the types of data being passed around, which reduces integration issues.
d) Seamless Integration with Modern JavaScript Frameworks
TypeScript works seamlessly with modern frontend frameworks like React, Angular, and Vue. In fact, Angular is built with TypeScript, and both React and Vue provide first-class TypeScript support. This makes it easier for developers to adopt TypeScript when working on projects that use these frameworks.
3. Common Patterns in TypeScript Development
a) Type Annotations and Interfaces
TypeScript allows you to specify the types of variables and function parameters explicitly. This makes your code more readable and self-documenting.
interface User {
id: number
name: string
email: string
}
function getUserInfo(user: User): string {
return `User: ${user.name} (${user.email})`
}
b) Generics for Reusable Components
Generics allow you to create reusable components that work with any data type. This is particularly useful in scenarios where you need to write generic functions or components.
function identity<T>(value: T): T {
return value
}
c) Union and Intersection Types
Union types let you specify multiple possible types for a value, while intersection types combine multiple types into one. This is useful for modeling more complex data structures.
type ID = number | string // Union type
interface Developer {
name: string
skills: string[]
}
interface Manager {
name: string
employees: string[]
}
type DevManager = Developer & Manager // Intersection type
4. How to Transition from JavaScript to TypeScript
Transitioning from JavaScript to TypeScript can be done incrementally, making it easy for teams to adopt. Here are some steps to help you get started:
a) Start with TypeScript in Strict Mode
Enable strict mode by setting strict: true
in your tsconfig.json
file. This enables all strict type-checking options and helps catch common mistakes early.
b) Gradual Migration
You don't have to rewrite your entire codebase in TypeScript immediately. You can start by renaming your JavaScript files (.js
) to TypeScript files (.ts
) and gradually add type annotations.
c) Use JSDoc Comments for Type Checking
For existing JavaScript code, you can use JSDoc comments to provide type annotations. This allows TypeScript to check types without requiring a full migration.
d) Leverage TypeScript’s Type Definition Files
If you're using third-party JavaScript libraries, you can often find TypeScript type definition files (.d.ts
) for them. This allows TypeScript to understand the types used by these libraries and provide better autocompletion and type checking.
5. Conclusion
TypeScript is revolutionizing the way developers build and maintain large JavaScript applications. With its powerful type system, excellent tooling support, and growing community, TypeScript is poised to become the standard for JavaScript development in 2024 and beyond.
By gradually adopting TypeScript, you can improve code quality, reduce bugs, and enhance collaboration among your team. Whether you're working on a new project or maintaining an existing one, TypeScript offers a range of benefits that can help you build more robust and scalable web applications.